Developing modern web apps using ASP.NET Core
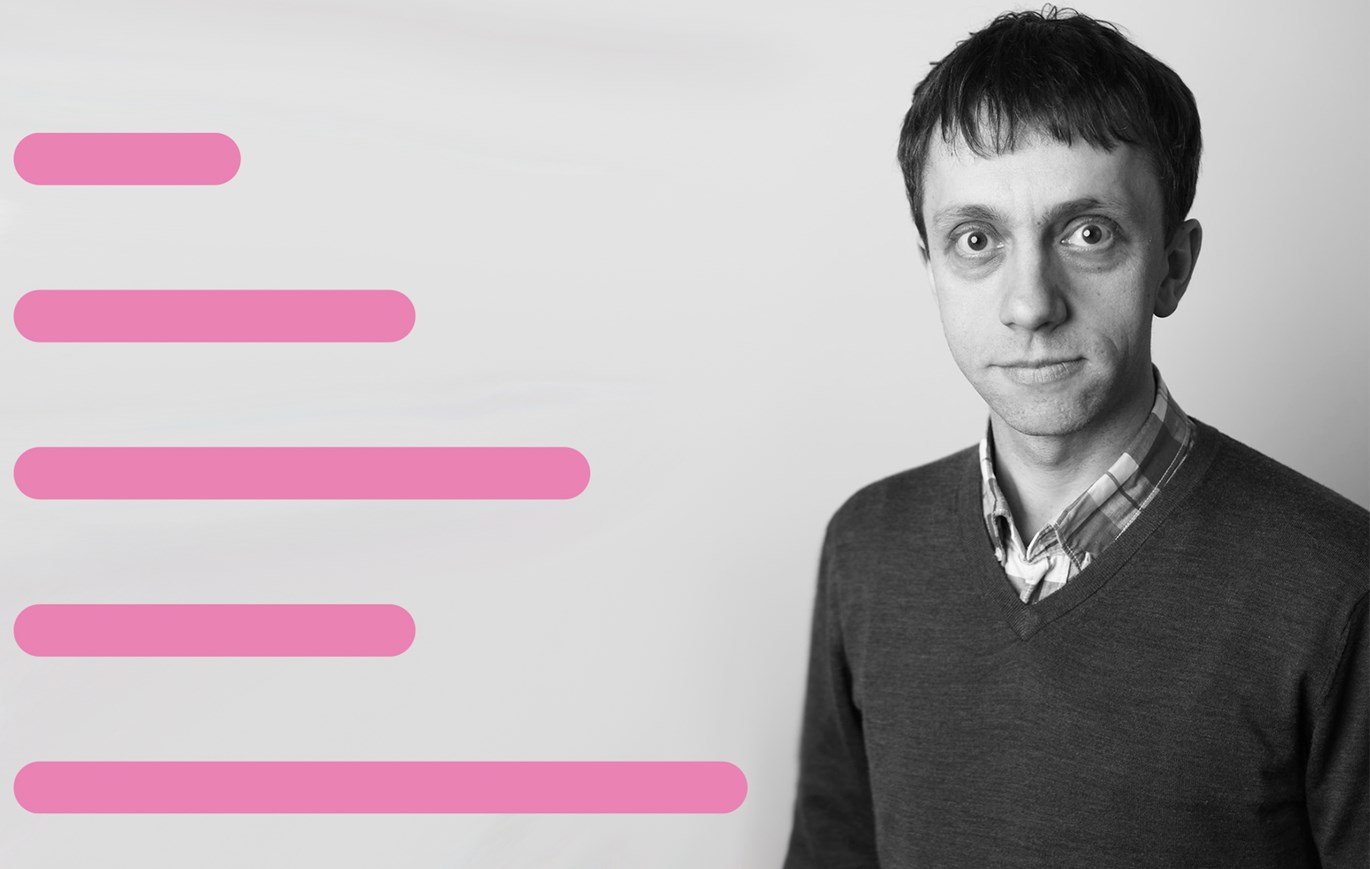
ASP.NET Core 8 is Microsoft’s premier platform to build web applications. In this course, you’ll get a solid understanding of ASP.NET Core, ASP.NET Core MVC, Blazor and Web API. This course uses .NET 8 and is fully updated for the new version of the underlying frameworks.
This course can act both as an introduction to students new to ASP.NET Core who want to get started with the newest version of the framework as well as an overview for seasoned ASP.NET developers willing to learn what’s new in this version of the framework. This course is also ideal for teams who are currently using older technologies such as ASP.NET WebForms or other web development technologies, not on the Microsoft stack.
After taking this course, developers will have a good understanding of the different options, covering the full stack, to create web apps using ASP.NET Core.
This course uses .NET 8 and Visual Studio 2022.
Target Audience and Prerequisites
This course is intended for web developers. The prerequisites are:
- A working knowledge of C#
- Basic web development (HTML, CSS, JavaScript)
- WebForms or other ASP.NET Experience is a plus but not required
About the trainer
Gill Cleeren has been working with ASP.NET since its inception in the early 2000s and has guided numerous projects based around .NET to a success. He has been teaching about the subject for many years and is also the author of several ASP.NET courses on Pluralsight, including the successful ASP.NET Core Fundamentals and ASP.NET Core Blazor Fundamentals. He’s an MVP and Microsoft Regional Director.
Detaljerad information
Understanding .NET 8 and ASP.NET Core 8
This first module will give you an overview of the .NET 8 platform and its components. You will understand the rational behind the .NET 8 philosophy and its cross-platform capabilities. We will also explore the different options that we have to create ASP.NET Core apps including Visual Studio 2022 and VS Code on Mac and Linux. Features such as Hot Reload will be explored here too and you’ll end this module with a well configured environment to get started on building your first ASP.NET Core app.
Topics:
- .NET 8 and ASP.NET Core 8
- Sharing code in .NET 8
- Setting up your environment using VS or VS Code (with C# Dev Kit)
- Working with Visual Studio 2022 and ASP.NET Core
Finding your way around ASP .NET Core
In this module, we will together create the File > new project and see what is getting generated. You’ll learn about the new files, workflow and configuration options that come with ASP.NET Core. You will also learn about the dependency injection system and the middleware request pipeline and how these can be configured from the Program.cs class. Along the way, you will learn about static files middleware and how this helps in terms of performance for your application, basic routing and other middleware. You’ll also learn about the different environments that are supported with ASP.NET Core. We will also touch on loading application settings as a means to configure your application.
Topics:
- Exploring a new ASP.NET Core project
- The Program.cs class
- Working with dependency injection
- Using middleware
- Creating an app from scratch and adding DI and middleware
Creating our first page
In this module, we’ll be building our first ASP.NET Core pages. We’ll start with basic configuration and add a first view, model and a controller. You’ll see how routing works as well as how we can allow users to authenticate with the site. After this module, you’ll already have a good understanding of how an ASP.NET Core MVC app is built and you’ll be able to identify its major building blocks such as controllers, routing and views.
Topics:
- Understanding MVC concepts
- Using the Model with mock data
- Creating a controller
- Adding views
- Using Layouts and view models
- Adding client-side packages
An introduction to EF Core
ASP.NET Core comes backed with support of a new version of Entity Framework Core 8. This new version of the framework has been redesigned from scratch to target .NET 8. In this module, we are exploring the new framework. We’ll see how it focuses entirely on code-first approaches and we will change our first pages now to use database interactions. You’ll learn about concepts such as the data context and you’ll start using LINQ to interact with the database.
Topics:
- Understand EF Core as an ORM
- Using the Database Context and unit of work
- Apply LINQ statements to work with the database
- Migrations and data seeding
Navigation and Routing
Routing is something specific to the ASP.NET Core MVC framework. In this chapter, you’ll learn about the options that are offered by the ASP.NET Core MVC framework to navigate to pages as well as creating well-formatted URLs for reasons of SEO.
Topics:
- Understand the concept of routing
- Tag helpers for navigation
- Routing constrains
Creating a Form
Forms are the basic building block of the web to accept input from users. Accepting incoming data from the browser can be a tedious and error prone task. That’s one of the reasons why ASP.NET Core comes with model binding. ASP.NET Core also comes with several controls to make it easy to validate the entered data, allowing for visualization of any entry errors made by the user. In this chapter, we will look at the model binding and its many capabilities, all while creating a form in the application.
Topics:
- Creating a form
- Using form tag helpers
- Using validation
An introduction to ASP.NET Identity
ASP.NET Core comes with ASP.NET Identity, a built-in framework that allows authenticating users from different sources. In this module, we’ll explore how we can authenticate users and how we can authorize them to perform actions within our site. We’ll use ASP.NET Core Identity which has many features built-in to perform authentication and authorization tasks.
Topics:
- Getting to know ASP.NET Core Identity
- Using the scaffolding to create login and registration forms
- Applying authorization to exclude pages from being accessed
Tackling security risks with ASP.NET Core
ASP.NET sites will be under attack, that’s for sure. We of course as the developers of the site need to make sure that we tackle risks before it’s deployed. In this module, we’ll see how we can tackle XSS, CSRF… as the most common security risks in our application.
Topics:
- Protecting against XSS
- Protecting against CSRF
Extending the views in ASP.NET Core
We at this point have several pages in our application and basically, creating simple pages will always be the same. In this chapter, we will extend on the view part. ASP.NET Core comes with tag helpers, we’ve already seen those. But we can create them ourselves and we’ll see why that would be useful. Next to tag helpers, view components can also be used to wrap functionality and reuse it. Partial views, also covered in this chapter, will do the same but on a simpler level. Understanding what to choose when is an important task too and that will be explained here as well.
Topics:
- Creating custom tag helpers
- Creating and using view components
- Using partial views
Advanced concepts in ASP.NET Core
In this chapter, we will cover in more detail several more advanced topics such as model binding (in more depth as it was already covered earlier), complex tag helpers, attribute routing, filters and background services. ASP.NET Core is very extensible and one of the often used extension points is custom middleware. All of these and more are covered in this chapter so that after completing this one, you are capable of creating a real-life ASP.NET Core application.
Topics:
- Complex tag helpers
- Custom middleware
- Different options for routing
- Creating background services
- Using and creating filters
Unit testing in ASP.NET Core
Building enterprise applications with ASP.NET Core without a good set of tests is far from a recommended approach. Through the use of unit tests, we check that the functionality we intended to create is effectively delivered and works as expected and continues to do so, even after making changes. In this chapter, we will explore how you can write unit tests for an ASP.NET Core MVC application using xUnit. We’ll learn how we can test different building blocks, of course including the controllers.
Topics:
- Understanding the why and how of unit tests
- Creating unit tests for the different building blocks of the application
Creating a REST API using ASP.NET Core
ASP.NET Core MVC now has unified the API and regular controller model, making the creation of APIs even simpler. APIs power the world and creating them is pretty easy with ASP.NET Core, especially since the knowledge you have gained so far in this course carries forward to creating APIs In this module, we are therefore focusing on the aspects that are closely related to RESTful Web APIs, built with the controller-based API model. Topics:
- Understanding the principles of REST APIs
- Creating an API with ASP.NET Core
- Working with response and status codes
Advanced concepts for creating APIs with ASP.NET Core
Once you know the basics of how to create an API, we’ll look at more advanced concepts such as using APIs (from clients), documenting APIs and authenticating with APIs. We’ll also explore how to create a Minimal API, which were introduced in ASP.NET Core 6.
Topics
- Working with APIs from the client
- Creating a Swagger endpoint
- Documenting an API
Understanding Blazor apps
Blazor introduces a radical new way for .NET developers to build client-side applications and since .NET 8, also server-side rendered applications. In this module, we will understand the concepts behind this new technology. You’ll understand the different hosting models which your Blazor code can run under and you’ll see the different files that make up a new project. You’ll create also your first Blazor component.
Topics:
- Understanding the different Blazor hosting models
- Exploring a new project in the different models
- Creating a first Blazor component
- Looking at Blazor Full-stack Web UI
Building components with Blazor
Blazor uses components to build the UI. Using APIs, we can connect these component with live data. And using the data binding features in Blazor, it’s easy to create data-driven UIs. This module will show us how we can build these in detail. We’ll also create a simple form, again driven by some of the built-in components in Blazor.
Topics:
- Data binding in Blazor components
- Accessing data behind a REST service
- Creating a form using built-in Blazor components
- Posting data to an endpoint from a Blazor application
Diagnostics and Logging in ASP.NET Core apps
Through middleware, ASP.NET Core applications can be configured to give diagnostic information to the users. Also, ASP.NET Core comes with a built-in logging system that we’ll explore in this module as well. We’ll also add some third-party logging providers here as well (Serilog).
Topics:
- Adding diagnostics middleware to your application
- Implementing logging in the application using built-in log providers
- Adding Serilog as log provider
Caching and performance in ASP.NET Core
Performance is vital for ASP.NET Core applications. In this chapter, we will learn how we can make use of the different ways of adding caching to our site, including in-memory caching, distributed caching and response caching. This way, we have several points in the application’s architecture where logging can be added to improve its performance.
Topics:
- Understanding the principles of caching
- Adding in-memory caching
- Using response caching
- Implementing a distributed cache
Kursen hålls på begäran - Kontakta oss för mer information.
Telefon: 08-562 557 50 E-post: kursbokning@cornerstone.se
Relaterat innehåll
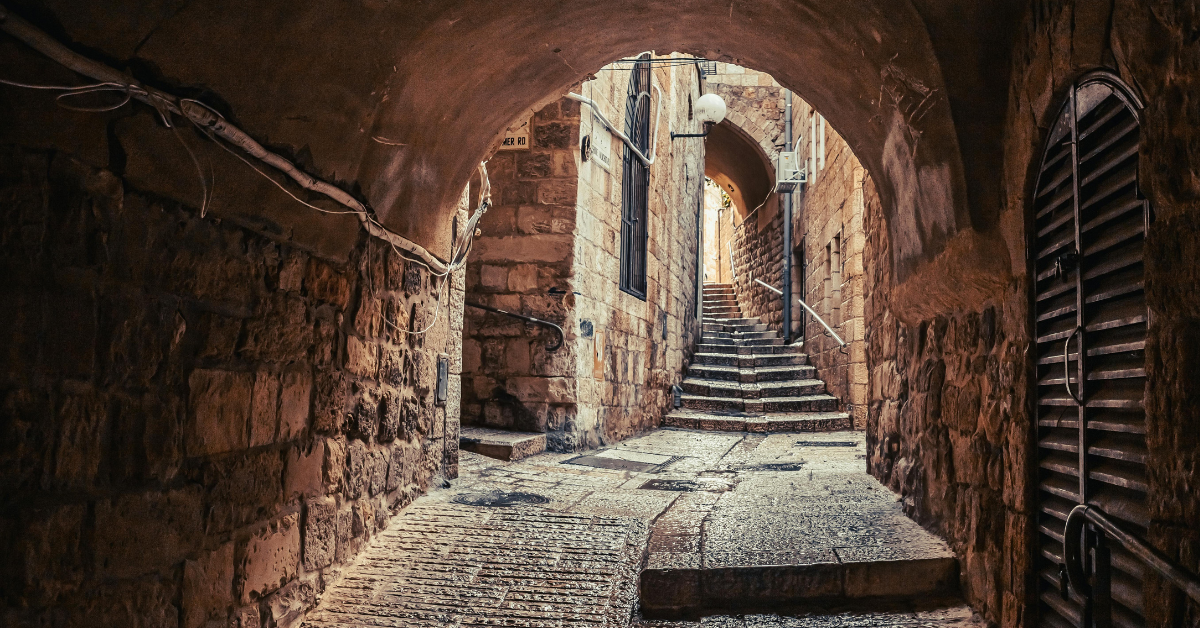
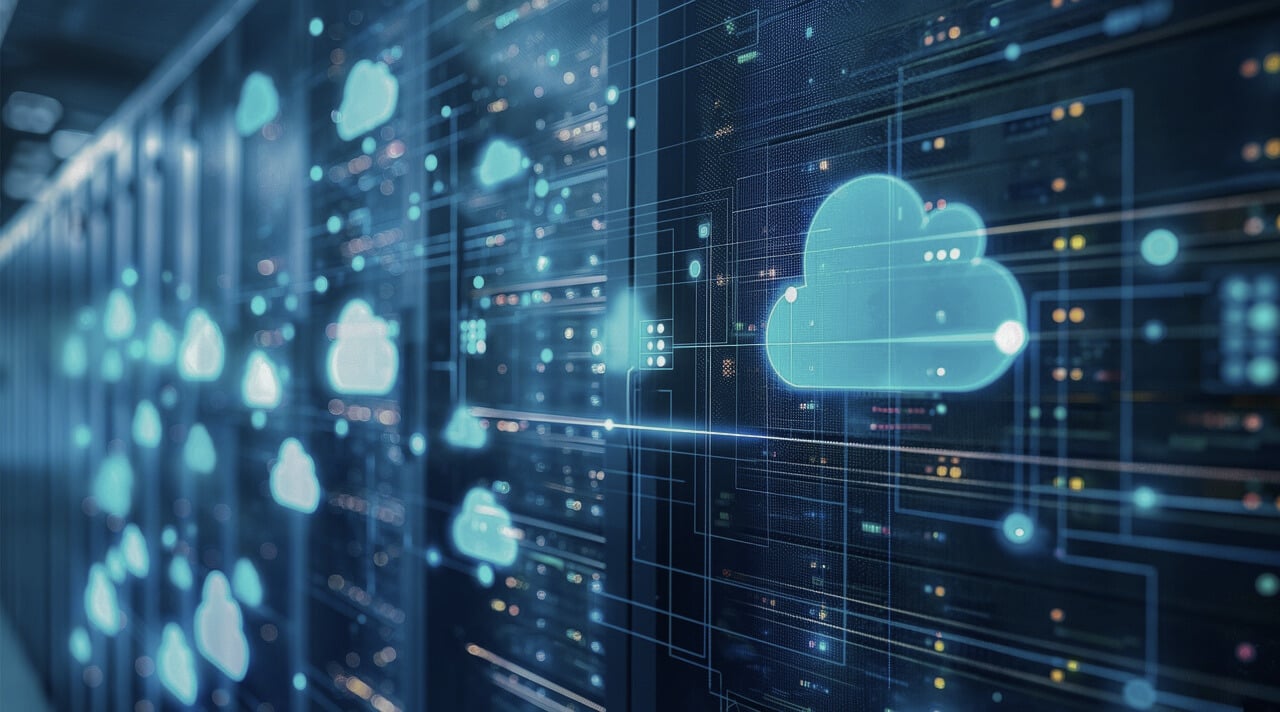